9 de septiembre de 2024
Hola, Mundo. ¿Echamos un tres en raya?
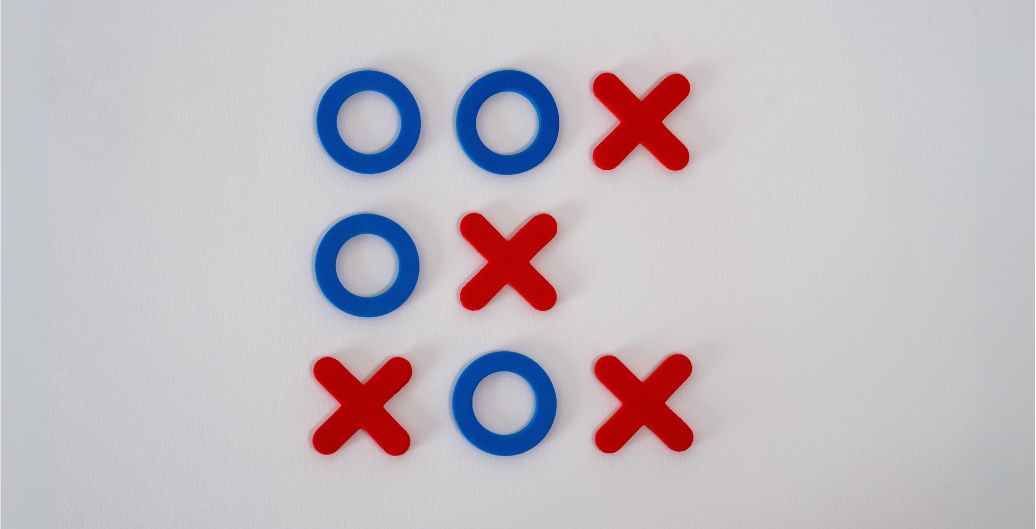
Doy inicio al blog de esta web, donde compartiré distintas cosas relacionadas con el desarrollo informático y mis descubrimientos en ese mundillo. Quizá la mejor forma de empezar sea echando una partida de Tres en Raya. Uno de los primeros proyectos que programé en C# fue este mítico juego, así que te dejo el código a continuación por si te apetece echar una partida.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace _3_EN_RAYA_DEFINITIVO
{
internal class Program
{
public static void Main(string[] args)
{
//CREACIÓN DE TABLERO
string[,] tablero =
{
{ "1" , "2" , "3"},
{ "4" , "5" , "6"},
{ "7", "8" , "9"}
};
//variable para la asignación de turnos
bool turno = true;
//Asignación inicial del estado de la partida
bool estadoPartida = true;
while (estadoPartida == true)
{
Console.Clear();
muestraTablero(tablero);
turnos(ref tablero, ref turno);
compruebaPartida(ref tablero, ref estadoPartida);
}
Console.ReadKey();
}
public static void muestraTablero(string[,] tablero)
{
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine(
$"{tablero[0, 0]}---{tablero[0, 1]}---{tablero[0, 2]}\n" +
$"{tablero[1, 0]}---{tablero[1, 1]}---{tablero[1, 2]}\n" +
$"{tablero[2, 0]}---{tablero[2, 1]}---{tablero[2, 2]}\n");
}
public static void turnos(ref string[,] tablero, ref bool turno)
{
bool turnoActual = turno;
string jugador = "";
if (turnoActual == true) jugador = "X";
else jugador = "O";
while (turno == turnoActual)
{
string marca = "";
if (jugador == "X") {
Console.WriteLine($"Turno del jugador {jugador}, introduzca el número con el que quiere jugar");
marca = Console.ReadLine();
Console.WriteLine($"Sigue el juego, pulsa enter para continuar.");
Console.ReadLine();
}
else
{
Random rnd = new Random();
marca = rnd.Next(1,10).ToString();
Console.WriteLine($"El jugador {jugador} ya ha jugado. Pulsa enter para continuar");
Console.ReadLine();
}
for (int i = 0; i < tablero.GetLength(0); i++)
{
for (int j = 0; j < tablero.GetLength(1); j++)
{
if (tablero[i, j] == marca)
{
tablero[i, j] = jugador;
if (turno == true) turno = false;
else turno = true;
}
}
}
}
}
public static void compruebaPartida(ref string[,] tablero, ref bool estadoTablero)
{
int contador = 0;
int valorComparativo = 1;
for (int i = 0; i < tablero.GetLength(0); i++)
{
for (int j = 0; j < tablero.GetLength(1); j++, valorComparativo++)
{
if (tablero[i, j] == valorComparativo.ToString())
{
contador++;
}
}
}
if (contador == 0) { estadoTablero = false; }
//COMPROBAR SI ALGUIEN HA GANADO
string[] letras = new string[8]
{
(tablero[0,0] + tablero [0,1] + tablero [0,2]),
(tablero[1,0] + tablero [1,1] + tablero [1,2]),
(tablero[2, 0] + tablero[2, 1] + tablero[2, 2]),
(tablero[0, 0] + tablero[1, 0] + tablero[2, 0]),
(tablero[0, 1] + tablero[1, 1] + tablero[2, 1]),
(tablero[0, 2] + tablero[1, 2] + tablero[2, 2]),
(tablero[0, 0] + tablero[1, 1] + tablero[2, 2]),
(tablero[0,2] + tablero [1,1] + tablero [2,0])
};
string resultadoGanador = "";
for (int i = 0; i < letras.Length; i++)
{
if (letras[i] == "XXX")
{
resultadoGanador += letras[i];
estadoTablero = false;
muestraTablero(tablero);
Console.WriteLine("El jugador X gana.");
}
else if (letras[i] == "OOO")
{
resultadoGanador += letras[i];
estadoTablero = false;
muestraTablero(tablero);
Console.WriteLine("El jugador O gana.");
}
}
//COMPROBAR EMPATES
if (resultadoGanador == "" && estadoTablero == false)
{
muestraTablero(tablero);
Console.WriteLine("Se ha producido un empate");
}
//preguntar si se quiere jugar de nuevo
if (estadoTablero == false)
{
nuevoIntento(ref estadoTablero, ref tablero);
}
}
public static void nuevoIntento(ref bool estadoPartida, ref string[,] tablero)
{
Console.WriteLine("¿Quieres jugar una nueva partida? (S/N)");
string nuevaPartida = Console.ReadLine().ToUpper();
if (nuevaPartida == "S")
{
estadoPartida = true;
int inicial = 1;
for (int i = 0; i < tablero.GetLength(0); i++)
{
for (int j = 0; j < tablero.GetLength(1); j++)
{
tablero[i, j] = inicial.ToString();
inicial++;
}
}
}
}
}
}